Getting started¶
This page is an overview of the features within pyGMS. Many of the methods shown here have plenty of configuration options. Please refer to the documentation of the individual methods to gain more information. An overview of the most important classes and their methods is given in API.
For installation instructreions see Installation. Generally, I recommend to use pyGMS together with Anaconda and jupyter.
Loading a model¶
Note
pyGMS currently only works with models that were saved in ASCII fromat.
To get started, import the GMS
class of pyGMS and
load a GMS model file.
In [1]: from pyGMS import GMS
In [2]: model = GMS('../../examples/model.fem')
Loading ../../examples/model.fem
Done!
Triangulating layers
Done!
You can display some information about to model using the
info
property:
In [3]: model.info
../../examples/model.fem
Number of layers 46
Number of unique layers 6
Points per layer 1881
Number of points 86526
xlim (200000.0, 1000000.0)
ylim (6200000.0, 7600000.0)
zlim (-102500.104684, 4736.80127)
To check whether it was loaded correctly, we can for example plot a profile:
In [4]: model.plot_topography();
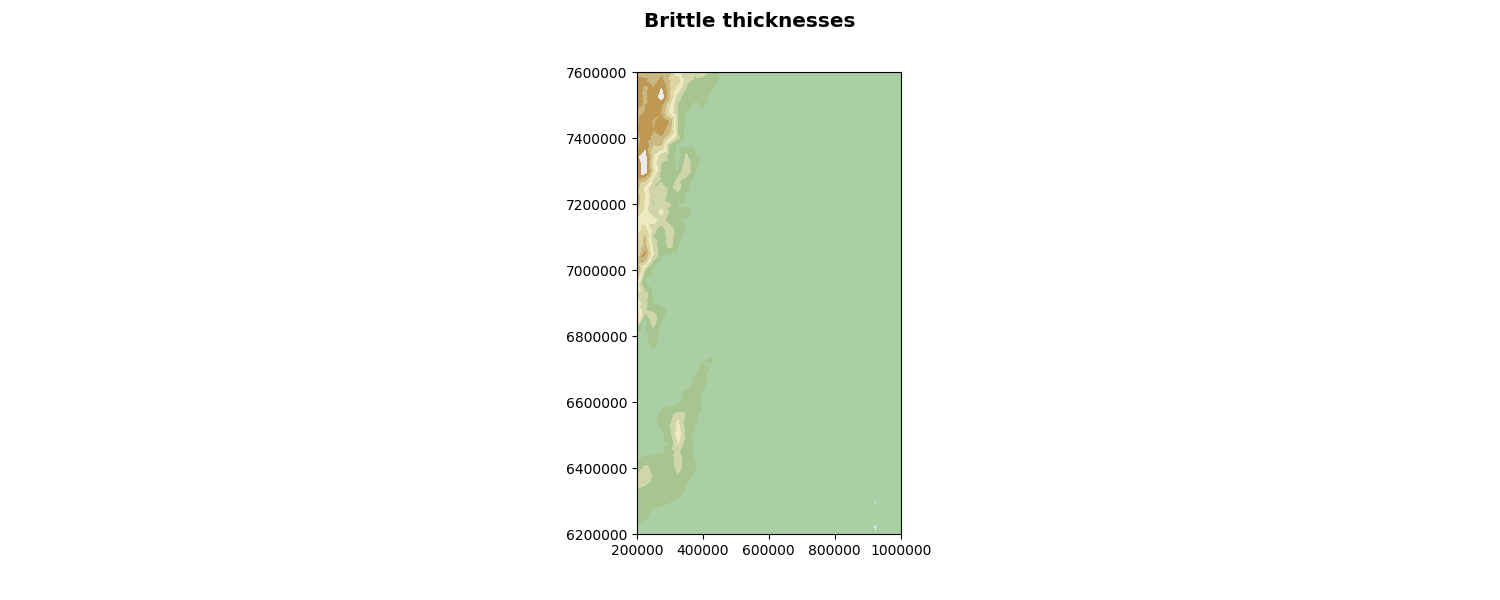
Note
The ; at the end of the command is only required for technical reasons within this documentation of pyGMS.
or list the layers:
In [5]: model.layer_dict_unique.items()
Out[5]: odict_items([(0, 'Sediments'), (5, 'UpperCrustRDP'), (15, 'UpperCrustPampia'), (25, 'LowerCrust'), (35, 'LithMantle'), (45, 'Base')])
Note
The layer references are stored as dictionaries that link the layer id to the layer name. The layer_dict_unique contains the primary layers, whereas the layer_dict also contains all refined layers.
Loading variables¶
To accelerate pyGMS, variables are not loaded into the model when initiating
an object. The available variable names are stored in the field_dict
of a
pyGMS model:
In [6]: model.field_dict.keys()
Out[6]: dict_keys(['X', 'Y', 'Z', 'RhoB', 'Tcond', 'Tspec', 'HeatGen', 'T'])
In order to add a variable, use the function
layer_add_var()
. In this case we add the
temperature variable T
:
In [7]: model.layer_add_var('T')